Configurable Test Double
The book has now been published and the content of this chapter has likely changed substanstially.Please see page 558 of xUnit Test Patterns for the latest information.
Also known as: Configurable Mock Object, Configurable Test Spy, Configurable Test Stub
Variation of: Test Double
How do we tell a Test Double what to return or expect?
Configure a reusable Test Double with the values to be returned or verified during the fixture setup phase of a test.
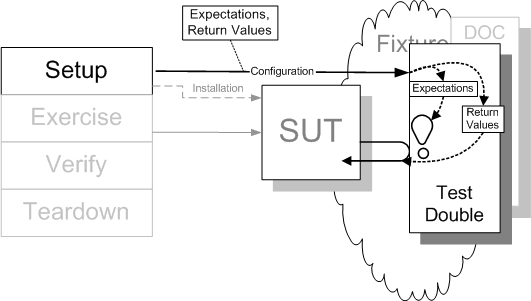
Sketch Configurable Test Double embedded from Configurable Test Double.gif
Some tests require unique values to be fed into the system under test (SUT) as indirect inputs or to be verified as indirect outputs of the SUT. This typically requires the use of Test Doubles (page X) as the conduit between the test and the SUT but the Test Double somehow needs to be told what values to return or verify.
A Configurable Test Double is a way to reduce Test Code Duplication (page X) by reusing a Test Double in many tests by configuring the values to be returned or expected at runtime.
How It Works
The Test Double is built with instance variables to hold the values to be returned to the SUT or to be the expected value of arguments to method calls. The test sets these variables during the setup phase of the test by calling the appropriate methods on the Test Double's interface. When the SUT calls the methods on the Test Double, it (the Test Double) uses the contents of the appropriate variable as the values to return or the expected value in assertions.
When To Use It
We can use a Configurable Test Double whenever we need similar but slightly different behavior in several tests that depend on Test Doubles and we want to avoid Test Code Duplication or when want to avoid having an Obscure Test (page X). (That is, if we must be able to see what values the Test Double is using as we read the test.) If we only expect a single usage of a Test Double, we can consider using a Hard-Coded Test Double (page X) instead if the extra effort and complexity of building a Configurable Test Double is not be warranted.
Implementation Notes
A Test Double is a Configurable Test Double because it needs to provide a way for the tests to configure it with values to return and/or method arguments to expect. Configurable Test Stubs (page X) and Test Spys (page X) only require a way to configure the responses to calls on their methods; configurable Mock Objects (page X) also require a way to configure their expectations (which methods should be called and with what arguments.)
There are many ways to build Configurable Test Doubles. Deciding on a particular implementation involves making two relataively independent decisions. The first relates to how the Configurable Test Double is to be configured while the second relates to how the Configurable Test Double is coded. There are two common ways to configure a Configurable Test Double. The most common way is to provide a Configuration Interface that is used only by the test to configure the values to be returned as indirect inputs and the expected values of the indirect outputs. The other alternative is to build the Configurable Test Double with two modes. The Configuration Mode is used during fixture setup to install the indirect inputs and expected indirect outputs by calling the methods of the Configurable Test Double with the expected arguments. Before the Configurable Test Double is installed, it is put into the normal ("usage" or "playback") mode.
The obvious way to build a Configurable Test Double is the Hand-Built Test Double but if we are fortunate, someone has already built a tool to generate a Configurable Test Double for us. Test Double generators come in two flavors; one flavor is a code generator while the other kind fabricates the object at run time. There have been several generations of "mocking" tools built by developers and several of these have been ported to other programming languages so it is worth checking http://xprogramming.com to see what is available in our programming language of choice. If the answer is "nothing", we can hand-code them ourself though this does take somewhat more effort.
Variation: Configuration Interface
A Configuration Interface is separate set of methods that the Configurable Test Double provides specifically for use by the test to set each value the Configurable Test Double needs to return or expect. The test simply calls these methods during the fixture setup phase of the Four-Phase Test (page X). The SUT uses the "other" methods on the Configurable Test Double; the "normal" interface. It isn't aware the Configuration Interface exists on the object it is delegating too.
Configuration Interfaces come in two flavors. Early toolkits like MockMaker generated a distinct method for each value we needed to configure. The collection of these setter methods made up the Configuration Interface. More recent toolkits like JMock provide a generic interface that is used to build an Expected Behavior Specification (see Behavior Verification on page X) that the Configurable Test Double interprets at run time. A well designed fluent interface can make the test much easier to read and understand.
Variation: Configuration Mode
We can avoid defining a separate set of methods to configure the Test Double by providing a Configuration Mode that the test uses to "teach" the Configuration Mode what to expect. At first glance, this means of configuring the Test Double can be confusing; why is the Test Method (page X) calling the methods of this other object before it is calling the methods it is exercising on the SUT? When we come to grips with the fact that we are doing a form of "record & playback", it all starts to make a bit more sense.
The main advantage of using a Configuration Mode is that it avoids creating a separate set of methods for configuring the Configurable Test Double since we reuse the same methods that the SUT will be calling. (We do have to provide a way to set the values to be returned by the methods and so we have at least one additional method to add.) On the flip side, each method that the SUT is expected to call now has two code paths through it, one for the Configuration Mode and another for the "Usage Mode".
Variation: Hand-Built Test Double
I've used Hand-Built Test Doubles in a lot of my examples because it is easier to see what is going on when we have actual, simple, concrete code to look at. This is the main advantage of using a Hand-Built Test Double and some people consider this important enough that they use Hand-Built Test Doubles exclusively. The other reason for using a Hand-Built Test Double is when there are no third-party toolkits available to use or if we are prevented from using them by project or corporate policy.
Variation: Statically Generated Test Double
The early third-party toolkits used code generators to create the code for the Statically Generated Test Doubles. The code then gets compiled and linked with our hand-written test code. Typically, we will store the code in our source code Repository[SCM]. Every time the interface of the target class changes, we need to regenerate the code for our Statically Generated Test Doubles. It may be advantageous to include this step as part of the automated build script to ensure that it really does happen whenever the interface changes.
Instantiating the Statically Generated Test Double is the same as instantiating a Hand-Built Test Double; we use the name of the generated class to construct the Configurable Test Double.
An interesting problem is what to do during refactoring. Suppose we changed the interface of the class we are replacing by adding an argument to one of the methods. Should we refactor the generated code? Or should we regenerate the Statically Generated Test Double after the code it replaces has been refactored? With modern refactoring tools, it may seem easier to refactor the generated code and the tests that use it in a single step but this may leave the Statically Generated Test Double without argument verification logic or variables for the new parameter. Therefore, we should regenerate the Statically Generated Test Double after the refactoring is finished to ensure that the refactored Statically Generated Test Double works properly and can be recreated by the code generator.
Variation: Dynamically Generated Test Double
Newer third-party toolkits generate Configurable Test Doubles at run time by using the reflection capabilities of the programming language to examine a class or interface and build an object that is capable of understanding all the calls to its methods. They may interpret the behavior specification at runtime or they may generate executable code but there is no code to generate and maintain or regenerate. The down side is simply that there is no code to look at and that really isn't a down side unless we are particularly suspicious or paranoid.
Note that most of the tools generate Mock Objects because they are the most fashionable and widely used. We can still use them as Test Stubs since they do provide a way of setting the value to be returned when a particular method is called. If we aren't particularly interested in verifying the methods being called or the arguments passed to them, most toolkits provide a way to specify "don't care" arguments. Since most toolkits generate Mock Objects, they typically don't provide a Retrieval Interface (see Test Spy).
Motivating Example
Here's a test that uses a Hard-Coded Test Double to give it control over the time:
public void testDisplayCurrentTime_AtMidnight_HCM() throws Exception { // fixture setup // Instantiate hard-code Test Stub: TimeProvider testStub = new MidnightTimeProvider(); // Instantiate SUT: TimeDisplay sut = new TimeDisplay(); // Inject Stub into SUT: sut.setTimeProvider(testStub); // exercise sut String result = sut.getCurrentTimeAsHtmlFragment(); // verify direct output String expectedTimeString = "<span class=\"tinyBoldText\">Midnight</span>"; assertEquals("Midnight", expectedTimeString, result); } Example HardCodedMockUsage embedded from java/com/clrstream/ex7/test/TimeDisplayTestSolution.java
This test is hard to understand without seeing the definition of the Hard-Coded Test Double. It is easy to see how this can lead to Mystery Guest (see Obscure Test) if it is not close at hand.
class MidnightTimeProvider implements TimeProvider { public Calendar getTime() { Calendar myTime = new GregorianCalendar(); myTime.set(Calendar.HOUR_OF_DAY, 0); myTime.set(Calendar.MINUTE, 0); return myTime; } } Example HardCodedMockDefn embedded from java/com/clrstream/ex7/test/TimeDisplayTestSolution.java
We can solve the Obscure Test problem by using a Self Shunt (see Hard-Coded Test Double) to make the Hard-Coded Test Double visible within the test:
public class SelfShuntExample extends TestCase implements TimeProvider { public void testDisplayCurrentTime_AtMidnight() throws Exception { // fixture setup TimeDisplay sut = new TimeDisplay(); // mock setup sut.setTimeProvider(this); // self shunt installation // exercise sut String result = sut.getCurrentTimeAsHtmlFragment(); // verify direct output String expectedTimeString = "<span class=\"tinyBoldText\">Midnight</span>"; assertEquals("Midnight", expectedTimeString, result); } public Calendar getTime() { Calendar myTime = new GregorianCalendar(); myTime.set(Calendar.MINUTE, 0); myTime.set(Calendar.HOUR_OF_DAY, 0); return myTime; } } Example SelfShunt embedded from java/com/clrstream/ex7/test/SelfShuntExample.java
Unfortunately, we will need to build a new Test Double for each Testcase Class (page X) and that results in Test Code Duplication.
Refactoring Notes
Refactoring a test from using a Hard-Coded Test Double into one that uses a third-party Configurable Test Double is pretty straightforward. We simply follow the directions provided with the toolkit to instantiate the Configurable Test Double and configure it with the same values as we used in the Hard-Coded Test Double. We may have to move some of the logic hard-coded within the Test Double into the Test Method and pass it in to the Test Double as part of the configuration steo.
Converting the actual Hard-Coded Test Double into a Configurable Test Double is a bit more complicated but not overly so if we only need to capture simple behavior. (For more complex behavior, we're probably better off examining one of the existing toolkits and porting it to our environment if it is not yet available.) First we need to introduce a way to set the values to be returned or expected. It is best to start by modifying the test to see how we want to interact with the Configurable Test Double. After instantiating it during the fixture setup part of the test, we then pass the test-specific values to the Configurable Test Double using the emerging Configuration Interface or Configuration Mode. Once we've seen how we want to use it, we can use an Introduce Field[JetBrains] refactoring to create the instance variables of the Configurable Test Double to hold each of the previously hard-coded values.
Example: Configuration Interface using Setters
In this example, I've shown how a test would use a simple hand-built Configuration Interface using Setters:
public void testDisplayCurrentTime_AtMidnight() throws Exception { // Fixture setup: // Test Double configuration TimeProviderTestStub tpStub = new TimeProviderTestStub(); tpStub.setHours(0); tpStub.setMinutes(0); // Instantiate SUT: TimeDisplay sut = new TimeDisplay(); // Test Double installation sut.setTimeProvider(tpStub); // exercise sut String result = sut.getCurrentTimeAsHtmlFragment(); // verify outcome String expectedTimeString = "<span class=\"tinyBoldText\">Midnight</span>"; assertEquals("Midnight", expectedTimeString, result); } Example MockTestWithProgramming embedded from java/com/clrstream/ex7/test/TimeDisplayTestSolution.java
The Configurable Test Double is implemented as follows:
class TimeProviderTestStub implements TimeProvider { // Configuration Interface: public void setHours(int hours) { // 0 is midnight; 12 is noon myTime.set(Calendar.HOUR_OF_DAY, hours); } public void setMinutes(int minutes) { myTime.set(Calendar.MINUTE, minutes); } // Interface used by SUT public Calendar getTime() { // @return The last time that was set: return myTime; } } Example ProgrammableTestDouble embedded from java/com/clrstream/ex7/test/TimeProviderTestStub.java
Example: Configuration Interface using Expression Builder
Now let us contrast that Configuration Interface with the one provided by the JMock framework. JMock generates Mock Objects dynamically and provides a generic interface for configuring the Mock Object in an intent-revealing style. Here's the same test converted to use JMock:
public void testDisplayCurrentTime_AtMidnight_JM() throws Exception { // Fixture setup: TimeDisplay sut = new TimeDisplay(); // Test Double configuration Mock tpStub = mock(TimeProvider.class); Calendar midnight = makeTime(0,0); tpStub.stubs().method("getTime").withNoArguments().will(returnValue(midnight)); // Test Double installation sut.setTimeProvider((TimeProvider) tpStub); // exercise sut String result = sut.getCurrentTimeAsHtmlFragment(); // verify outcome String expectedTimeString = "<span class=\"tinyBoldText\">Midnight</span>"; assertEquals("Midnight", expectedTimeString, result); } Example JMockStubUsage embedded from java/com/clrstream/ex7/test/TimeDisplayTestSolution.java
Note how we've had to move some of the logic to construct the time to return into the Testcase Class because there is no way to do it in the mocking framework; we've used a Test Utility Method (page X) to construct the time to be returned. This next example shows a configurable Mock Object complete with multiple expected parameters:
public void testRemoveFlight_JMock() throws Exception { // fixture setup FlightDto expectedFlightDto = createAnonRegFlight(); FlightManagementFacade facade = new FlightManagementFacadeImpl(); // mock configuration Mock mockLog = mock(AuditLog.class); mockLog.expects(once()).method("logMessage") .with(eq(helper.getTodaysDateWithoutTime()), eq(Helper.TEST_USER_NAME), eq(Helper.REMOVE_FLIGHT_ACTION_CODE), eq(expectedFlightDto.getFlightNumber())); // mock installation facade.setAuditLog((AuditLog) mockLog.proxy()); // exercise facade.removeFlight(expectedFlightDto.getFlightNumber()); // verify assertFalse("flight still exists after being removed", facade.flightExists( expectedFlightDto.getFlightNumber())); // verify() method called automatically by JMock } Example JMockUsage embedded from java/com/clrstream/ex8/test/FlightManagementFacadeTestSolution.java
Note how the Expected Behavior Specification is built by calling expression-building methods like expects, once and method to describe how the Configurable Test Double should be used and what it should return. JMock supports specifying much more sophisticated behavior (such as multiple calls to the same method with different arguments and return values) than does our hand-built Configurable Test Double.
Example: Configuration Mode
In this example, I've converted the test to use a Mock Object with a Configuration Mode.
public void testRemoveFlight_ModalMock() throws Exception { // fixture setup FlightDto expectedFlightDto = createAnonRegFlight(); // mock configuration (in config mode) ModalMockAuditLog mockLog = new ModalMockAuditLog(); mockLog.logMessage(helper.getTodaysDateWithoutTime(), Helper.TEST_USER_NAME, Helper.REMOVE_FLIGHT_ACTION_CODE, expectedFlightDto.getFlightNumber()); mockLog.enterPlaybackMode(); // mock installation FlightManagementFacade facade = new FlightManagementFacadeImpl(); facade.setAuditLog(mockLog); // exercise facade.removeFlight(expectedFlightDto.getFlightNumber()); // verify assertFalse("flight still exists after being removed", facade.flightExists( expectedFlightDto.getFlightNumber())); mockLog.verify(); } Example ProgrammingModeUsage embedded from java/com/clrstream/ex8/test/FlightManagementFacadeTestSolution.java
Note how the test is calling the methods on the Configurable Test Double during the fixture setup phase. If we weren't aware that this was a Configurable Test Double mock, we might find this confusing at first glance. The most obvious clue is the call to the method enterPlaybackMode which tells the Configurable Test Double to stop saving expected values and to start asserting on them.
The Configurable Test Double that this test is using is implemented like this:
private int mode = record; public void enterPlaybackMode() { mode = playback; } public void logMessage( Date date, String user, String action, Object detail) { if (mode == record) { Assert.assertEquals("Only supports 1 expected call", 0, expectedNumberCalls); expectedNumberCalls = 1; expectedDate = date; expectedUser = user; expectedCode = action; expectedDetail = detail; } else { Assert.assertEquals("Date", expectedDate, date); Assert.assertEquals("User", expectedUser, user); Assert.assertEquals("Action", expectedCode, action); Assert.assertEquals("Detail", expectedDetail, detail); } } Example ProgrammingModeDefn embedded from java/com/clrstream/ex8/test/ModalMockAuditLog.java
Note the if statement that checks whether we are in record or playback mode. Because this simple hand-built Configurable Test Double only supports a single value to store, I've used a Guard Assertion (page X) to fail the test if it tries to record more than one call to this method. The rest of the then clause saves the parameters into variables that it uses as the expected values of the Equality Assertions (see Assertion Method on page X) in the else clause.
Copyright © 2003-2008 Gerard Meszaros all rights reserved