Guard Assertion
The book has now been published and the content of this chapter has likely changed substanstially.Please see page 490 of xUnit Test Patterns for the latest information.
How do we avoid Conditional Test Logic?
Replace an if statement in a test with an assertion that fails the test if not satisfied.
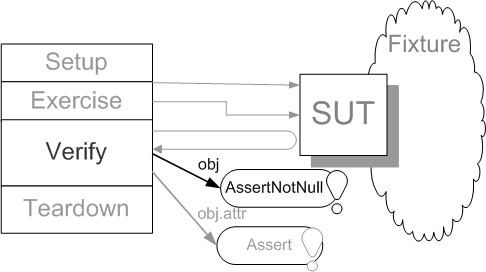
Sketch Guard Assertion embedded from Guard Assertion.gif
Some verification logic may fail because information returned by the system under test (SUT) is not initialized as expected. When a test encounters an unexpected problem, it may result in a test error rather than a test failure. While the Test Runner (page X) does its best to provide useful diagnostic information, the test automater can often do better by checking for the particular condition and reporting it explicitly.
A Guard Assertion is a good way to do this without introducing Conditional Test Logic (page X).
How It Works
Tests either pass or fail. We fail tests by calling Assertion Methods (page X) that stop the test from executing further if the assertion's condition is not met. Conditional Test Logic that is used to avoid executing assertions when they would cause test errors can be replaced by assertions that fail the test instead. This also acts as good documentation that we expect the condition of the Guard Assertion to be true. A failure of the Guard Assertion makes it very clear that some condition we expected to be true was not; this avoids the effort of inferring it from conditional logic.
When To Use It
We should use a Guard Assertion whenever we want to avoid executing statements because they would cause an error if they were executed when some condition related to values returned by the SUT are not true. We do this instead of putting an if then else fail code construct around the sensitive statements. Normally, this is done before the verify outcome phase of our Four-Phase Test (page X).
Variation: Shared Fixture State Assertion
When using a Shared Fixture (page X) a Guard Assertion can also be useful right at the beginning of the test to verify that the Shared Fixture satisifies the test's needs. It also makes it clearer to the test reader what parts of the Shared Fixture this test actually uses and that improves the likelihood of achieving Tests as Documentation (see Goals of Test Automation on page X).
Implementation Notes
We can use Stated Outcome Assertions (see Assertion Method) and Equality Assertions (see Assertion Method) as Guard Assertions that fail the test and prevent execution of other statements that would cause test errors.
Motivating Example
Consider the following example:
public void testWithConditionals() throws Exception { String expectedLastname = "smith"; List foundPeople = PeopleFinder.findPeopleWithLastname(expectedLastname); if (foundPeople != null) { if (foundPeople.size() == 1) { Person solePerson = (Person) foundPeople.get(0); assertEquals( expectedLastname,solePerson.getName()); } else { fail("list should have exactly one element"); } } else { fail("list is null"); } } Example ConditionalAssertion embedded from java/com/xunitpatterns/guardassertion/Example.java
In this example there are plenty of conditional statements for the author to get wrong; things like writing (foundPeople == null) instead of (foundPeople != null). In C-based languages, one might mistakenly use = instead of == and that would result in the test always passing!
Refactoring Notes
We can use a Replace Nested Conditional with Guard Clauses[Fowler] refactoring to transform this spider web of Conditional Test Logic into a nice linear sequence of statements. (In a test, even a single conditional statement is considered too much and hence "nested"!) We can use Stated Outcome Assertions to check for null object references and Equality Assertions to verify the number of objects in the collection. If each assertion is satisfied, the test proceeds. If they are not satisfied, the test ends in failure before the next statement is reached.
Example: Simple Guard Assertion
This simplified version of the test replaces all the conditionals with assertions. This test is shorter than the original and much easier to read.
public void testWithoutConditionals() throws Exception { String expectedLastname = "smith"; List foundPeople = PeopleFinder.findPeopleWithLastname(expectedLastname); assertNotNull("found people", foundPeople); assertEquals( "number of people", 1, foundPeople.size() ); Person solePerson = (Person) foundPeople.get(0); assertEquals( "last name", expectedLastname, solePerson.getName() ); } Example GuardAssertion embedded from java/com/xunitpatterns/guardassertion/Example.java
We now have a single linear execution path through this Test Method (page X); that should improve our confidence in the correctness of this test immensely!
Example: Shared Fixture Guard Assertion
Here's an example of a test that depends on a Shared Fixture. If the state of the fixture is modified by a previous test (or even this test in a previous test run), our SUT could return unexpected results. It might take a fair bit of effort to determine that the problem is with the test's preconditions rather than a bug in the SUT. We can avoid all this trouble by making the assumptions of this test explicit through the use of Guard Assertions during the fixture lookup phase of the test.
public void testAddFlightsByFromAirport_OneOutboundFlight_GA() throws Exception { // Fixture Lookup: List flights = facade.getFlightsByOriginAirport( ONE_OUTBOUND_FLIGHT_AIRPORT_ID ); // Guard Assertion on fixture contents: assertEquals( "# flights precondition", 1, flights.size()); FlightDto firstFlight = (FlightDto) flights.get(0); // Exercise System BigDecimal flightNum = facade.createFlight( firstFlight.getOriginAirportId(), firstFlight.getDestAirportId()); // verify outcome: FlightDto expFlight = (FlightDto) firstFlight.clone(); expFlight.setFlightNumber( flightNum ); List actual = facade.getFlightsByOriginAirport( firstFlight.getOriginAirportId()); assertExactly2FlightsInDtoList( "Flights at origin", firstFlight, expFlight, actual); } Example SharedFixtureGuardAssertion embedded from java/com/clrstream/ex6/services/test/SharedFixtureFlightManagementFacadeTest.java
We now have a way to know that the assumptions were violated without extensive debugging! This is another way we achieve Defect Localization (see Goals of Test Automation) (this time the defect is in the tests.)
Copyright © 2003-2008 Gerard Meszaros all rights reserved