Inline Setup
The book has now been published and the content of this chapter has likely changed substanstially.How do we construct the Fresh Fixture?
Each Test Method creates it's own Fresh Fixture by calling the appropriate constructor methods to build exactly the test fixture it requires.
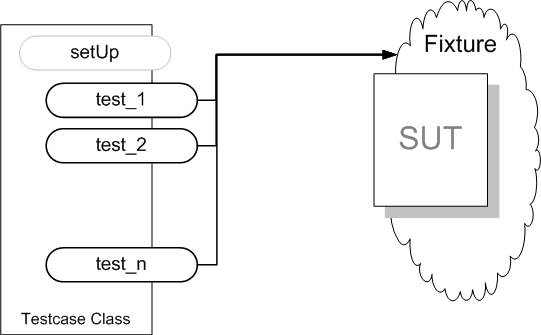
Sketch Inline Setup embedded from Inline Setup.gif
To execute an automated test, we require a text fixture that is well understood and completely deterministic. We can use the Fresh Fixture (page X) approach to build a Minimal Fixture (page X) for the use of this one test. Setting up the test fixture inline in each test is the most obvious way to build it.
How It Works
Each Test Method (page X) sets up it's own test fixture by directly calling whatever system under test (SUT) code is required to construct exactly the test fixture it requires. We put the code that creates the fixture, the first phase of the Four-Phase Test (page X), at the top of each Test Method.
When To Use It
We can use Inline Setup when the fixture setup logic is very simple and straight-forward. As soon as the fixture setup gets at all complex, we should consider using Delegated Setup (page X) or Implicit Setup (page X) for part of all of the fixture setup.
We can also use Inline Setup when writing a first draft of tests and we haven't yet figured out what part of the fixture setup will be repeated from test to test. This is an example of applying the "Red-Green-Refactor" process pattern to the tests themselves but we need to be careful when we refactor the tests to ensure that we don't break the tests in ways that are undetectable.
A third occasion to use Inline Setup is when refactoring obtuse fixture setup code. A first step may be to use Inline Method[Fowler] refactorings on all Creation Methods (page X) and the setUp method. Then we can try using Extract Method[Fowler] refactoring to define a new set of Creation Method that are more reusable or intent-revealing.
Implementation Notes
In practice, most fixture setup logic will include a mix of styles including Inline Setup building on top of Implicit Setup or Delegated Setup interspersed with Inline Setup.
Example: Inline Setup
Here's an example of simple inline setup. Everything each Test Method needs for exercising the SUT is included inline.
public void testStatus_initial() { // inline setup: Airport departureAirport = new Airport("Calgary", "YYC"); Airport destinationAirport = new Airport("Toronto", "YYZ"); Flight flight = new Flight(flightNumber, departureAirport, destinationAirport); // Exercise SUT & verify outcome assertEquals(FlightState.PROPOSED, flight.getStatus()); // tearDown: // garbage-collected } public void testStatus_cancelled() { // inline setup: Airport departureAirport = new Airport("Calgary", "YYC"); Airport destinationAirport = new Airport("Toronto", "YYZ"); Flight flight = new Flight( flightNumber, departureAirport, destinationAirport); flight.cancel(); // still part of setup // Exercise SUT & verify outcome assertEquals(FlightState.CANCELLED, flight.getStatus()); // tearDown: // garbage-collected } Example InlineSetup embedded from java/com/clrstream/ex6/services/test/SetupStyles.java
Refactoring Notes
Inline Setup is normally the starting point for refactoring, not the end goal. But, sometimes we find ourselves with tests that are too hard to understand because of all the stuff happening behind the scenes, a form of Mystery Guest (see Obscure Test on page X). Or, we may find ourselves modifying the previously setup fixture in many of the tests.
These are both indications it may be time to refactor our test class into multiple classes based on the fixture they build. First, we use Inline Method refactoring to inline all the code to result in Inline Setup. Then we reorganize the tests using a Extract Class[Fowler] refactoring and finally we use a series of Extract Method refactorings to define a more understandable set of fixture setup methods.
Copyright © 2003-2008 Gerard Meszaros all rights reserved