Mock Object
The book has now been published and the content of this chapter has likely changed substanstially.Please see page 544 of xUnit Test Patterns for the latest information.
Variation of: Test Double
How do we implement Behavior Verification for indirect outputs of the SUT?
How can we verify logic independently when it depends on indirect inputs from other software components?
Replace an object the system under test (SUT) depends on with a test-specific object that verifies it is being used correctly by the SUT.
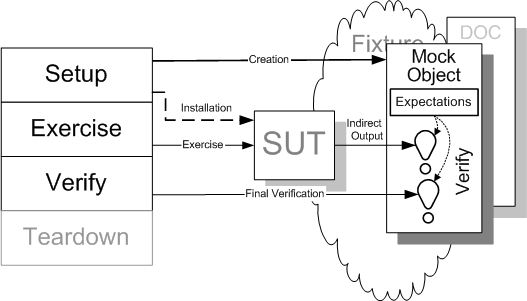
Sketch Mock Object embedded from Mock Object.gif
In many circumstances, the environment or context in which the system under test (SUT) operates very much influences the behavior of the SUT. In other cases, we must peer "inside"(Technically, the SUT is whatever software we are testing and doesn't include anything it depends on so "inside" is a misnomer. It is better to think of the depended-on component (DOC) that is the destination of indirect outputs as being "behind" the SUT.) the SUT to be able to determine whether the expected behavior has occurred.
A Mock Object is a powerful way to implement Behavior Verification (page X) while avoiding Test Code Duplication (page X) between similar tests by delegating the job of verifying the indirect outputs of the SUT entirely to a Test Double (page X).
How It Works
First, we define a Mock Object that implements the same interface as an object on which the SUT depends. Then, during the test, we configure the Mock Object with the values with which it should respond to the SUT and the method calls (complete with expected arguments) to expect from the SUT. Before exercising the SUT, we install the Mock Object so that the SUT uses it instead of the real implementation. When called during SUT execution, the Mock Object compares the actual arguments received with the expected arguments using Equality Assertions (see Assertion Method on page X) and fails the test if they don't match. The test need not do any assertions at all!
When To Use It
We can use a Mock Object as an observation point when we need to do Behavior Verification to avoid having an Untested Requirement (see Production Bugs on page X) caused by an inability to observe side-effects of invoking methods on the SUT. Another common use is endoscopic testing [ET] or need-driven development. We don't need to use a Mock Object when we are doing State Verification (page X) but we might use a Test Stub (page X) or Fake Object (page X). Note that test drivers have found other uses for the Mock Object toolkits but many of these are actually examples of using a Test Stub rather than a Mock Object.
To use a Mock Object, we need to be able to predict the value of most or all arguments of the method calls before we exercise the SUT. We should not use a Mock Object if a failed assertion cannot be reported effectively back to the Test Runner (page X). This may be the case if the SUT is running inside a container that catches and eats all exceptions. In these cases we may be better off using a Test Spy (page X) instead.
Mock Objects (especially those created using dynamic mocking tools) often use the equals method of the various objects being compared. If our test-specific equality is different from how the SUT would interpret equals, we may not be able to use Mock Object or we may be forced to add an equals method where we didn't need one. This smell is called Equality Pollution (see Test Logic in Production on page X). Some implementations of Mock Object avoid this problem by allowing us to specify the "comparator" to be used in the Equality Assertions.
Mock Objects can be either "strict" or "lenient" (sometimes called "nice".) A "strict" Mock Object fails the test if the calls are received in a different order than was specified when the Mock Object was programmed. A "lenient" Mock Object tolerates out-of-order calls.
Implementation Notes
Tests written using Mock Objects look different from more traditional tests because all the expected behavior must be specified before the SUT is exercised. This makes them harder to write and to understand for test automation neophytes. This factor may be enough to cause us to prefer writing our tests using Test Spys.
The standard Four-Phase Test (page X) is altered somewhat when we use Mock Objects in that the fixture setup phase of the test is broken down into three specific activities and the result verification phase more or less disappears except for the possible presence of a call to the "final verification" method at the end of the test.
-
Fixture set up:
- Test constructs Mock Object.
- Test configures Mock Object. This is omitted for Hard-Coded Test Doubles (page X).
- Test installs Mock Object into SUT.
-
Exercise SUT:
- SUT calls Mock Object; Mock Object does assertions.
-
Result verification:
- Test calls "final verification" method.
-
Fixture tear down:
- no impact
Let's examine these differences a bit more closely:
Construction
As part of the fixture setup phase of our Four-Phase Test, we must construct the Mock Object that will replace the substitutable dependency. Depending on what tools are available in our programming language, we can either hand build the Mock Object class, use a code generator to create a Mock Object class or use a dynamically-generated Mock Object.
Configuration with Expected Values
This step is not needed with a Hard-Coded Test Double such as an Inner Test Double (see Hard-Coded Test Double). The Mock Object toolkits available in many members of the xUnit family typically create Configurable Mock Objects (page X) so we need to configure the Mock Object with the expected method calls (and their parameters) as well as the values to be returned by any functions. (Some Mock Object frameworks allow us to disable verification of the method calls or just their parameters.) We typically do this before we install the test double.
Installation
Of course, we must have a way of installing a Test Double into the SUT to be able to use a Mock Object. We can use whichever substitutable dependency pattern the SUT supports. A common approach in the test-driven development community is Dependency Injection (page X) while more traditional development may favor Dependency Lookup (page X).
Usage
When the SUT calls the methods of the Mock Object, they compare the method call (method name plus arguments) with the expectations. If the method call is unexpected or the arguments are incorrect, the assertion fails the test immediately. If the call is expected but came out of sequence, a strict Mock Object fails the test immediately while a lenient Mock Object notes that the call was received and carries on. Missed calls are detected in the Final Verification method.
If the method call has any outgoing parameters, the Mock Object needs to return or updated something to allow the SUT to continue executing the test scenario. This can either be hard-coded or it may have been configured at the same time as the expectations. This behavior is the same as for Test Stubs except that we typically return happy path values.
Final Verification
Most of the result verification occurs inside the Mock Object as it is called by the SUT. The Mock Object will fail the test if the methods are called with the wrong arguments or if methods are called unexpectedly. But what happens if expected method calls are never received by the Mock Object? The Mock Object may have trouble detecting that the test is over and it is time to check for unfulfilled expectations. Therefore, we need to ensure that the final verification method is called. Some Mock Object toolkits have found a way to invoke this method automatically by including the call in the tearDown method but many toolkits still require us to remember to call the final verification method ourselves.
Motivating Example
The following test verifies the basic functionality of creating a flight. But it does not verify the indirect outputs of the SUT, namely, the SUT is expected to log each time a flight is created along with day/time and username of the requester.
public void testRemoveFlight() throws Exception { // setup FlightDto expectedFlightDto = createARegisteredFlight(); FlightManagementFacade facade = new FlightManagementFacadeImpl(); // exercise facade.removeFlight(expectedFlightDto.getFlightNumber()); // verify assertFalse("flight should not exist after being removed", facade.flightExists( expectedFlightDto.getFlightNumber())); } Example UntestedRequirementTest embedded from java/com/clrstream/ex8/test/FlightManagementFacadeTest.java
Refactoring Notes
Verification of indirect outputs can be added to existing tests by using a Replace Dependency with Test Double (page X) refactoring. This involves adding code to the fixture setup logic of our test to create the Mock Object, configuring it with the and the expected method calls, arguments and values to be returned and installing it using whatever substitutable dependency mechanism is provided by the SUT. At the end of the test, we add a call to the "final verification" method if our Mock Object framework requires one.
Example: Mock Object (Hand-Coded)
In this improved version of the test, mockLog is our Mock Object. The method setExpectedLogMessage is used to program it with the expected log message. The statement facade.setAuditLog(mockLog) installs the Mock Object using the Setter Injection (see Dependency Injection) test double installation pattern. And finally, the verify() method ensures that the call to logMessage() was actually made at all.
public void testRemoveFlight_Mock() throws Exception { // fixture setup FlightDto expectedFlightDto = createAnonRegFlight(); // mock configuration ConfigurableMockAuditLog mockLog = new ConfigurableMockAuditLog(); mockLog.setExpectedLogMessage( helper.getTodaysDateWithoutTime(), Helper.TEST_USER_NAME, Helper.REMOVE_FLIGHT_ACTION_CODE, expectedFlightDto.getFlightNumber()); mockLog.setExpectedNumberCalls(1); // mock installation FlightManagementFacade facade = new FlightManagementFacadeImpl(); facade.setAuditLog(mockLog); // exercise facade.removeFlight(expectedFlightDto.getFlightNumber()); // verify assertFalse("flight still exists after being removed", facade.flightExists( expectedFlightDto.getFlightNumber())); mockLog.verify(); } Example ActiveMockObject embedded from java/com/clrstream/ex8/test/FlightManagementFacadeTestSolution.java
All this was made possible through the use of the following Mock Object. I've chosen to use a hand-built Mock Object and in the interest of space, I'll just show you what the logMessage method looks like:
public void logMessage( Date actualDate, String actualUser, String actualActionCode, Object actualDetail) { actualNumberCalls++; Assert.assertEquals("date", expectedDate, actualDate); Assert.assertEquals("user", expectedUser, actualUser); Assert.assertEquals("action code", expectedActionCode, actualActionCode); Assert.assertEquals("detail", expectedDetail,actualDetail); } Example HandCodedActiveMockMethod embedded from java/com/clrstream/ex8/test/ConfigurableMockAuditLog.java
Note that the Assertion Methods are being called as static methods. In JUnit, this is required because the Mock Object is not a subclass of TestCase so it does not inherit the assertion methods from Assert. Other members of the xUnit family may provide different mechanisms to access the Assertion Methods. For example, NUnit provides them only as static methods on the Assert classs so even Test Methods (page X) need to access them this way. Test::Unit, the xUnit family member for the Ruby programming language, provides them as mixins.
Example: Mock Object (Dynamically Generated)
The last example used a hand-coded Mock Object. Most members of the xUnit family have dynamic Mock Object frameworks available. Here's the same test rewritten using JMock:
public void testRemoveFlight_JMock() throws Exception { // fixture setup FlightDto expectedFlightDto = createAnonRegFlight(); FlightManagementFacade facade = new FlightManagementFacadeImpl(); // mock configuration Mock mockLog = mock(AuditLog.class); mockLog.expects(once()).method("logMessage") .with(eq(helper.getTodaysDateWithoutTime()), eq(Helper.TEST_USER_NAME), eq(Helper.REMOVE_FLIGHT_ACTION_CODE), eq(expectedFlightDto.getFlightNumber())); // mock installation facade.setAuditLog((AuditLog) mockLog.proxy()); // exercise facade.removeFlight(expectedFlightDto.getFlightNumber()); // verify assertFalse("flight still exists after being removed", facade.flightExists( expectedFlightDto.getFlightNumber())); // verify() method called automatically by JMock } Example JMockUsage embedded from java/com/clrstream/ex8/test/FlightManagementFacadeTestSolution.java
Note how JMock provides a "fluent" Configuration Interface (see Configurable Test Double) that allows us to specify the expected method calls in a fairly readable fashion. JMock also allows us to specify the comparator to be used by the assertions; in this case, the calls to eq cause the default equals method to be called.
Further Reading
Just about every book on automated testing using xUnit has something to say about Mock Objects so I won't list them here. As you are reading other books keep in mind that the term Mock Object is often used to refer to a Test Stub and sometimes even to Fake Objects. The appendix Mocks, Fakes, Stubs and Dummies contains a more thorough comparison of the terminology used in various books and articles.
Copyright © 2003-2008 Gerard Meszaros all rights reserved