Test-Specific Subclass
The book has now been published and the content of this chapter has likely changed substanstially.Also known as: Test-Specific Extension
How can we make code testable when we need to access private state of the SUT?
Add methods that expose the state or behavior needed by the test to a subclass of the SUT.
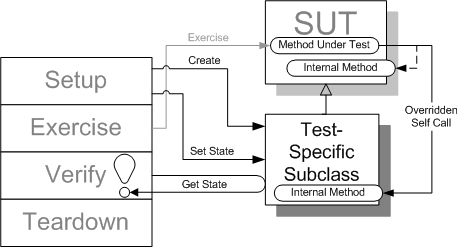
Sketch Test-Specific Subclass embedded from Test-Specific Subclass.gif
If the system under test (SUT) was not designed specifically to be testable, we may find that the test cannot get access to state that it must initialize or verify at some point in the test.
A Test-Specific Subclass is a simple yet very powerful way to open up the SUT for testing purposes without modifying the code of the SUT itself.
How It Works
Define a subclass of the SUT and add methods that modify the behavior of the SUT just enough to make it testable by implementing control points and observation points. This typically involves exposing instance variables using setters and getters or maybe adding a method to put the SUT into a specific state without moving through its entire lifecycle.
Since the Test-Specific Subclass would be packaged up with the tests that use it, the use of a Test-Specific Subclass does not change how the SUT is seen by the rest of the application.
When To Use It
We should use a Test-Specific Subclass whenever we need to modify the SUT to improve its testability but doing so directly would result in Test Logic in Production (page X). We can use a Test-Specific Subclass for a number of purposes but what they all have in common is that they improve testability by letting us get at the insides of the SUT more easily. Test-Specific Subclass can be a double-edged sword because by breaking encapsulation, it allows us to tie our tests even more closely to the implementation and that can result in Fragile Tests (page X). Here are some common uses of Test-Specific Subclass:
Variation: State Exposing Subclass
If we are doing State Verification (page X), we can subclass the SUT (or some component of it) so that we can see the internal state of the SUT for use in Assertion Methods (page X). Usually, this involves adding accessor methods for internal instance variables. We may also allow setting of the state by the test as a way to avoid Obscure Tests (page X) caused by Obscure Setup (see Obscure Test) logic.
Variation: Behavior Exposing Subclass
If we want to test the individual steps of a complex algorithm individually, we can subclass the SUT to expose the private methods that implement the Self-Calls[WWW]. Most languages do not allow relaxing visibility of a method so we often have to use a different name in the Test-Specific Subclass and make a call to the superclass' method.
Variation: Behavior Modifying Subclass
If the SUT contains some behavior that we do not want to have occur when testing, we can override whatever method implements the behavior with an empty method body. This works best when the SUT uses Self-Calls (or a Template Method[GOF]) to delegate the steps of an algorithm to methods on itself or subclasses.
Variation: Test Double Subclass
Also known as: Subclassed Test Double
We can ensure a Test Double (page X) is type-compatible with the component it is to replace by making it a subclass of that component. This may be the only way we can build a Test Double that the compiler will accept when variables are statically-typed using concrete classes. (We should not have to do this with dynamically typed languages like Ruby, Python, Perl or JavaScript.) We then override any methods whose behavior we want to change and add any methods we require to to make it a Configurable Test Double (page X) if we so desire.
Unlike the Behavior Modifying Subclass, this variation does not just "tweak" the behavior of the SUT (or a part thereof) but replaces it entirely with canned behavior.
Variation: Substituted Singleton
Also known as: Subclassed Singleton, Substitutable Singleton
A special case of Test Double Subclass is the Substituted Singleton. This is used when we want to replace a depended-on component (DOC) with a Test Double and the SUT does not support Dependency Injection (page X) or Dependency Lookup (page X).
Implementation Notes
The main challenge with using a Test-Specific Subclass are:
- Feature Granularity: ensuring that any behavior we want to override or expose is in a small enough method. This is enabled through copious use of small methods and Self-Calls.
- Feature Visibility: ensuring that subclasses can access attributes and behavior of the SUT class. This is primarily an issue in statically-typed languages like Java, C# and C++; dynamically-typed languages typically do not enforce visibility.
As with Test Doubles, we must be careful to ensure that we do not replace any of the behavior we are actually trying to test.
In languages that support class extensions without the need for subclassing (e.g. Smalltalk, Ruby, JavaScript and other dynamic languages), Test-Specific Subclass can be implemented as a class extension in the test package. We need to be aware, however, whether or not the extensions will make it into production; doing so would introduce Test Logic in Production.
Visibility of Features
In languages that enforce scope (visibility) of variables and methods, we may need to change the visibility of the variables to allow subclasses to access them. While this is indeed a change to the SUT code, it would typically be considered much less intrusive or misleading than changing the visibility to public (thereby allowing any code in the application to access the variables) or adding the test-specific methods directly to the SUT.
For example, in Java, we might change the visibility of instance variables from private to protected to allow the Test-Specific Subclass to access them. Similarly, we might change the visibility of methods to allow the Test-Specific Subclass to call them.
Variation: Self Delegation
Long methods are hard to test because they often bring too many dependencies into play. Short methods tend to be much simpler to test because they only do one thing. Self Delegation is a simple way to reduce the size of methods. We delegate parts of an algorithm to other methods implemented on the same class. This allows us to test these methods independently and we can also test that the calling method calls them in the right sequence by overriding these methods in a Test Double Subclass (see Test-Specific Subclass on page X).
Self Delegation is a part of good object-oriented code design by keeping methods small and focused on implementing a single responsibility of the SUT. We can use Self Delegation whenever we are doing test-driven development and have control over the design of the SUT. We may find that we need to introduce Self Delegation when we encounter long methods where some parts of the algorithm depend on things we do not want to exercise (such as database calls.) This is especially likely when the SUT is built using a Transaction Script[PEAA] architecture. Self Delegation can be retrofitted easily using the Extract Method[Fowler] refactoring supported by most modern IDE's.
Motivating Example
The test in this example is non-deterministic because it depends on the time. Our SUT is an object that formats the time for display as part of a web page. It gets the time by asking a Singleton called TimeProvider which gets the time from a calendar object that it gets from the container.
public void testDisplayCurrentTime_AtMidnight() throws Exception { // Setup SUT: TimeDisplay theTimeDisplay = new TimeDisplay(); // Exercise SUT: String actualTimeString = theTimeDisplay.getCurrentTimeAsHtmlFragment(); // Verify outcome: String expectedTimeString = "<span class=\"tinyBoldText\">Midnight</span>"; assertEquals( "Midnight", expectedTimeString, actualTimeString); } public void testDisplayCurrentTime_AtOneMinuteAfterMidnight() throws Exception { // Setup SUT: TimeDisplay actualTimeDisplay = new TimeDisplay(); // Exercise SUT: String actualTimeString = actualTimeDisplay.getCurrentTimeAsHtmlFragment(); // Verify outcome: String expectedTimeString = "<span class=\"tinyBoldText\">12:01 AM</span>"; assertEquals( "12:01 AM", expectedTimeString, actualTimeString); } Example NondeterministicTests embedded from java/com/xunitpatterns/dft/test/TimeDisplayTest.java
These tests rarely pass and never in the same test run! The code within the SUT looks like this:
public String getCurrentTimeAsHtmlFragment() { Calendar timeProvider; try { timeProvider = getTime(); } catch (Exception e) { return e.getMessage(); } // etc.} protected Calendar getTime() { return TimeProvider.getInstance().getTime(); } Example SelfDelegation embedded from java/com/xunitpatterns/dft/TimeDisplay.java
The code for the Singleton is:
public class TimeProvider { protected static TimeProvider soleInstance = null; protected TimeProvider() {}; public static TimeProvider getInstance() { if (soleInstance==null) soleInstance = new TimeProvider(); return soleInstance; } public Calendar getTime() { return Calendar.getInstance(); } } Example SubclassableSingleton embedded from java/com/xunitpatterns/dft/TimeProvider.java
Refactoring Notes
The nature of the refactoring to introduce a Test-Specific Subclass depends on why we are using one. When using a Test-Specific Subclass to expose "private parts" of the SUT or override undesirable parts of its behavior, we merely create the Test-Specific Subclass as a subclass of the SUT and create an instance the Test-Specific Subclass to exercise within our Test Methods (page X) or setUp method.
If, however, we are using the Test-Specific Subclass to replace a DOC of the SUT then we need to use a Replace Dependency with Test Double (page X) refactoring to tell the SUT to use our Test-Specific Subclass instead of the real DOC.
In either case, we either override existing or add additional methods to the Test-Specific Subclass using our language-specific capabilities (e.g. subclassing or mixins) as required by our tests.
Example: Behavior Modifying Subclass (Test Stub)
Because the SUT uses a Self-Call to the getTime method to ask the TimeProvider for the time, we have an opportunity to use a Subclassed Test Double to control the time. (This is enable by the fact that getTime was defined to be protected; we would not be able to do this if it was private.) Based on this idea we can take a stab at writing our tests as follows (I have only shown one here):
public void testDisplayCurrentTime_AtMidnight() { // Fixture setup: TimeDisplayTestStubSubclass tss = new TimeDisplayTestStubSubclass(); TimeDisplay sut = tss; // Test Double configuration tss.setHours(0); tss.setMinutes(0); // exercise sut String result = sut.getCurrentTimeAsHtmlFragment(); // verify outcome String expectedTimeString = "<span class=\"tinyBoldText\">Midnight</span>"; assertEquals( expectedTimeString, result ); } Example TestStubSubclassUsage embedded from java/com/xunitpatterns/dft/test/TimeDisplayTssTest.java
Note how I have used the Test-Specific Subclass class for the variable that receives the instance of the SUT; this is so that the methods of the Configuration Interface (see Configurable Test Double) defined on the Test-Specific Subclass are visible to the test. (I could have used a Hard-Coded Test Double (page X) subclass instead but that would have required a different Test-Specific Subclass for each time I want to test with. Each subclass would simply hard-code the return value of the getTime method.) For documentation purposes, I have then assigned it to the variable sut; this is a safe cast because the Test-Specific Subclass class is a subclass of the SUT class. This also helps avoid the Mystery Guest (see Obscure Test) problem caused by hard-coding an important indirect input of our SUT inside the Test Stub (page X).
Now that we have seen how it will be used, it is a simple matter to implement the Test-Specific Subclass:
public class TimeDisplayTestStubSubclass extends TimeDisplay { private int hours; private int minutes; // The overridden method: protected Calendar getTime() { Calendar myTime = new GregorianCalendar(); myTime.set(Calendar.HOUR_OF_DAY, this.hours); myTime.set(Calendar.MINUTE, this.minutes); return myTime; } /* * The Configuration Interface: */ public void setHours(int hours) { this.hours = hours; } public void setMinutes(int minutes) { this.minutes = minutes; } } Example TestStubSubclass embedded from java/com/xunitpatterns/dft/test/TimeDisplayTestStubSubclass.java
No rocket science here; we just had to implement the methods used by the test.
Example: Behavior Modifying Subclass (Substituted Singleton)
Suppose our getTime method was declared to be private(This means it cannot be seen or overridden by a subclass.) or static, final or sealed, etc.(This prevents a subclass from overriding its behavior.)? This would prevent us from overriding its behavior in our Test-Specific Subclass. What could we do to address our Nondeterministic Tests (see Erratic Test on page X)?
Since the design uses a Singleton[GOF] to provide the time, a simple solution is to replace the Singleton during test execution with a Test Double Subclass. We can do this as long as it is possible for a subclass to access its soleInstance variable. We use the Introduce Local Extension[Fowler] refactoring (specifically, the subclass variant of it) to create the Test-Specific Subclass. Writing the tests first helps us understand what interface we want to implement.
public void testDisplayCurrentTime_AtMidnight() { TimeDisplay sut = new TimeDisplay(); // Install test Singleton TimeProviderTestSingleton timeProvideSingleton = TimeProviderTestSingleton.overrideSoleInstance(); timeProvideSingleton.setTime(0,0); // Exercise SUT: String actualTimeString = sut.getCurrentTimeAsHtmlFragment(); // Verify outcome: String expectedTimeString = "<span class=\"tinyBoldText\">Midnight</span>"; assertEquals( expectedTimeString, actualTimeString ); } Example SubclassedSingletonTest embedded from java/com/xunitpatterns/dft/test/TimeDisplayTestSolution.java
Now that we have a test that uses the Substituted Singleton, we can proceed to implement it by subclassing the Singleton and defining the methods the tests will use.
public class TimeProviderTestSingleton extends TimeProvider { private Calendar myTime = new GregorianCalendar(); private TimeProviderTestSingleton() {}; // Installation Interface: static TimeProviderTestSingleton overrideSoleInstance() { // we could save the real instance first, but we won't! soleInstance = new TimeProviderTestSingleton(); return (TimeProviderTestSingleton) soleInstance; } // Configuration Interface used by the test: public void setTime(int hours, int minutes) { myTime.set(Calendar.HOUR_OF_DAY, hours); myTime.set(Calendar.MINUTE, minutes); } // Usage Interface used by the client: public Calendar getTime() { return myTime; } } Example SingletonTestDouble embedded from java/com/xunitpatterns/dft/test/TimeProviderTestSingleton.java
Note that the Test Double is a subclass of the real component and that it has overridden the instance method called by the clients of the Singleton.
Example: Behavior Exposing Subclass
Suppose we wanted to test the getTime method:
public void testGetTime_default() { // create SUT: TimeDisplayBehaviorExposingTss tsSut = new TimeDisplayBehaviorExposingTss(); // exercise SUT: // Want to do: // Calendar time = sut.getTime(); // Have to do: Calendar time = tsSut.callGetTime(); // verify outcome assertEquals( defaultTime, time ); } Example BehaviorExposingSubclassUsage embedded from java/com/xunitpatterns/dft/test/TimeDisplayTssTest.java
Because getTime is protected and our test is in a different package from the TimeDisplay class, our test cannot call this method. We could try making our test a subclass of TimeDisplay or we could put it into the same package as TimeDisplay. Unfortunately, both of these solutions come with baggage and may not always be possible.
A more general solution is to expose the behavior using Behavior Exposing Subclass. We can do this by defining a Test-Specific Subclass and adding a public method that calls this method.
public class TimeDisplayBehaviorExposingTss extends TimeDisplay { public Calendar callGetTime() { return super.getTime(); } } Example BehaviorExposingSubclass embedded from java/com/xunitpatterns/dft/test/TimeDisplayBehaviorExposingTss.java
Example: Defining Test-Specific Equality (Behavior Modifying Subclass)
Here is an example of a very simple test that is failing because the object we are passing to assertEquals does not implement test-specific equality. That is, the default equals method is returning false even though our test considers the two objects equals.
protected void setUp() throws Exception { oneOutboundFlight = findOneOutboundFlightDto(); } public void testGetFlights_OneFlight() throws Exception { // Exercise System List flights = facade.getFlightsByOriginAirport( oneOutboundFlight.getOriginAirportId()); // Verify Outcome assertEquals("Flights at origin - number of flights: ", 1, flights.size()); FlightDto actualFlightDto = (FlightDto)flights.get(0); assertEquals("Flight DTOs at origin", oneOutboundFlight, actualFlightDto); } Example DefaultEqualityTest embedded from java/com/clrstream/ex6/services/test/FlightManagementFacadeTssTest.java
One option is to write a Custom Assertion (page X). Another option is to use a Test-Specific Subclass to add a more appropriate definition of equality for our test purposes alone. We can change our fixture set up code slightly to create the Test-Specific Subclass as our Expected Object (see State Verification).
private FlightDtoTss oneOutboundFlight; private FlightDtoTss findOneOutboundFlightDto() { FlightDto realDto = helper.findOneOutboundFlightDto(); return new FlightDtoTss(realDto) ; } Example MakeTestSpecificSubclassDto embedded from java/com/clrstream/ex6/services/test/FlightManagementFacadeTssTest.java
Finally, we implement the Test-Specific Subclass copying and comparing only those fields that we want to use for our test-specific equality.
public class FlightDtoTss extends FlightDto { public FlightDtoTss(FlightDto realDto) { this.destAirportId = realDto.getDestinationAirportId(); this.equipmentType = realDto.getEquipmentType(); this.flightNumber = realDto.getFlightNumber(); this.originAirportId = realDto.getOriginAirportId(); } public boolean equals(Object obj) { FlightDto otherDto = (FlightDto) obj; if (otherDto == null) return false; if (otherDto.getDestAirportId()!= this.destAirportId) return false; if (otherDto.getOriginAirportId()!= this.originAirportId) return false; if (otherDto.getFlightNumber()!= this.flightNumber) return false; if (otherDto.getEquipmentType() != this.equipmentType ) return false; return true; } } Example TestSpecificEquality embedded from java/com/clrstream/ex6/services/test/FlightDtoTss.java
This assumes that we have a reasonable toString implementation on our base class that prints out the values of the fields being compared. This is needed because assertEquals will use that when the equals method returns false. Otherwise, we will have no idea of why the objects are considered unequal.
Example: State Exposing Subclass
Suppose we have the following test that requires a Flight to be in a particular state:
protected void setUp() throws Exception { super.setUp(); scheduledFlight = createScheduledFlight(); } Flight createScheduledFlight() throws InvalidRequestException{ Flight newFlight = new Flight(); newFlight.schedule(); return newFlight; } public void testDeschedule_shouldEndUpInUnscheduleState() throws Exception { scheduledFlight.deschedule(); assertTrue("isUnsched", scheduledFlight.isUnscheduled()); } Example StateRequiringTest embedded from java/com/clrstream/ex3/solution/flightbooking/domain/flightstate/fixturetests/TestScheduledFlight.java
Setting up the fixture for this test requires we call the method schedule on the flight.
public class Flight{ protected FlightState currentState = new UnscheduledState(); /** * Transitions the Flight from the <code>unscheduled</code> * state to the <code>scheduled</code> state. * @throws InvalidRequestException when an invalid state * transition is requested */ public void schedule() throws InvalidRequestException{ currentState.schedule(); } } Example PrivateState embedded from java/com/clrstream/ex3/solution/flightbooking/domain/Flight.java
The Flight class uses the State[GOF] pattern and delegates handling of the schedule method to whatever State object is currently referenced by currentState. This test will fail during fixture setup if schedule does not work yet on the default content of currentState. We can get around this by using a State Exposing Subclass that provides a method to move directly into the state thereby making this an Independent Test (see Principles of Test Automation on page X).
public class FlightTss extends Flight { public void becomeScheduled() { currentState = new ScheduledState(); } } Example StateExposingSubclass embedded from java/com/clrstream/ex3/solution/flightbooking/domain/flightstate/fixturetests/FlightTss.java
By introducing a new method becomeScheduled on the Test-Specific Subclass we have ensured that we will not accidently override any existing behavior of the SUT.Now all we have to do is instantiate the Test-Specific Subclass in our test instead of the base class by modifying our Creation Method (page X) .
Flight createScheduledFlight() throws InvalidRequestException{ FlightTss newFlight = new FlightTss(); newFlight.becomeScheduled(); return newFlight; } Example StateExposingSubclassTest embedded from java/com/clrstream/ex3/solution/flightbooking/domain/flightstate/fixturetests/StateExposingSubclass.java
Note how we still declare that we are returning an instance of the Flight class when we are in fact returning an instance of the Test-Specific Subclass that has the additional method.
Copyright © 2003-2008 Gerard Meszaros all rights reserved